PowerShell offers different tools to use to read files into arrays. In this article, we will discuss two of them:
- Get-Content cmdlet and
- Import-Csv cmdlet
After discussing the general functionality of these cmdlets, we will cover some examples that show how to control how the data from files are loaded and how to access items from the resulting arrays.
Using Get-Content to Read File into Array
The Get-Content cmdlet is used to get the contents of a file into a PowerShell array where each array element contains a line of content from the file. The general syntax of the cmdlet is given by (showing a few parameters only):
Get-Content -Path <string> -Total Count <number> -Tail <number> – Delimiter <number> -ReadCount <number> -Raw -Wait -Include <string> -Exclude <string> -Filter <string>
Where -Path is the location of the file we want to read. We go through the other parameters in the examples.
Loading File into PowerShell Array using Import-Csv
Import-Csv cmdlet loads the CSV file into a table-like custom array where each column in the CSV becomes a property of the custom array, and the row values become the property values.
Unlike Get-Content, which is purposed for different file types, Import-Csv is mainly used to load CSV files. However, we can repurpose it to load various file types.
Import-Csv has the following syntax (showing 3 relevant parameters only):
Import-Csv -Path <path> -Delimiter <value_separator> -Header <custom_headers>
Examples – Using Get-Content and Import-Csv Cmdlets
This Section covers how to use the two cmdlets to load two files – a text file saved as file1.txt and a CSV file stored as employees.csv.
Note: The contents of a given example may depend on the results of a previous example.
File: file1.txt
This is the content of file1.txt Lorem Ipsum is not random It is a long established fact There are many variations.
File: employees.csv
name|id Tomasz|011 Ammon|015
Example 1: Getting contents of a file using Get-Content
1 2 3 |
$data = Get-Content -Path ".\file1.txt" $data $data.Count # Count the number of lines. |
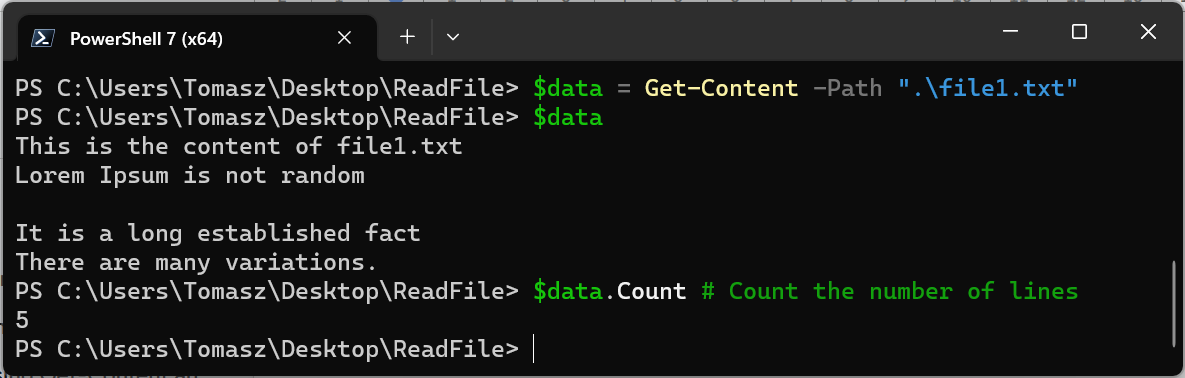
The Get-Content in the code snippet above loads the contents of the file1.txt file into the $data variable. There are 5 lines in the file; therefore, $data array will contain 5 elements as shown by $data.Count.
Example 2: Accessing values of the resulting array
Note that PowerShell scripting uses zero-based indexing – indexing starting from zero. Therefore, the first element is at index 0, the second is at index 1, and so on.
Here are some code lines showing how to access elements of the array.
1 2 3 4 5 |
$data[0] # first element $data[2] # third element $data[-1] # last element $data[1..3] # second to the fourth element $data[0,1,4] # First, second and 5th |
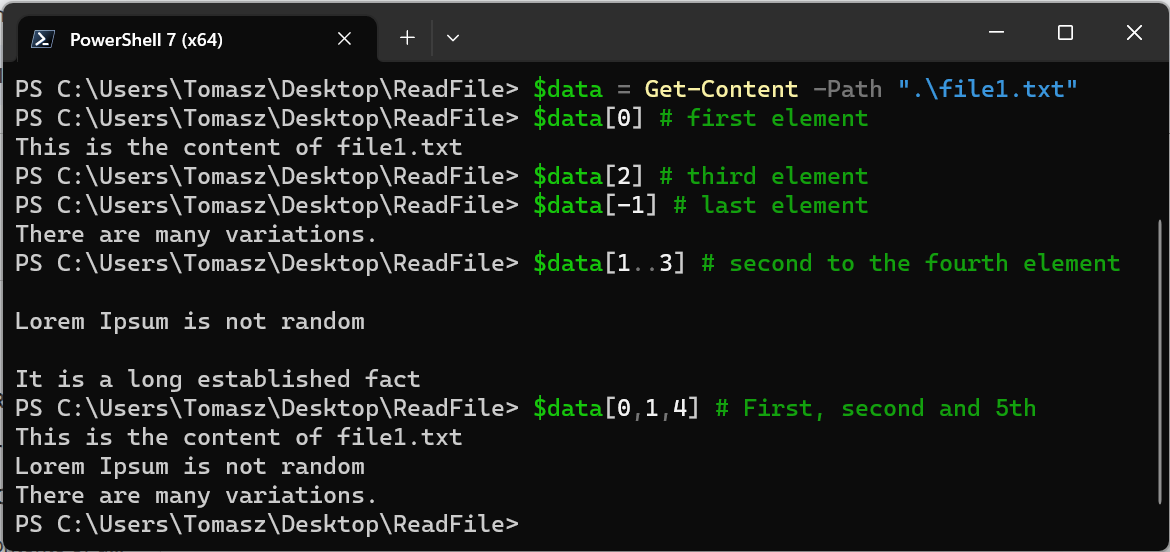
Example 3: Loading Specific lines from the file
Based on the indexing knowledge from Example 2, we can load specific lines from the file with something like this:
1 2 |
# For loading the first line (Get-Content -Path ".\file1.txt")[0] |
# For loading the second through the 5th line.
1 |
(Get-Content -Path ".\file1.txt")[1..4] |
Example 4: Reading the first x lines or the last x lines
This is where -TotalCount and -Tail parameters come in handy – the -TotalCount x to read x lines on the top and -Tail x to read x lines from the bottom.
1 2 3 4 |
# Reading the first 3 lines Get-Content -Path ".\file1.txt" -TotalCount 3 # Loading the last 2 lines Get-Content -Path ".\file1.txt" -Tail 2 |
Example 5: Loading lines containing a specific substring
If we want to read lines containing a given substring, we must pipe the Get-Content output into Where-Object to evaluate the condition. In the following example, we want to load lines with the substring “is” using regular expressions (documentation).
Get-Content -Path “.\file1.txt” | Where-Object {$_ -Like “*is*”}
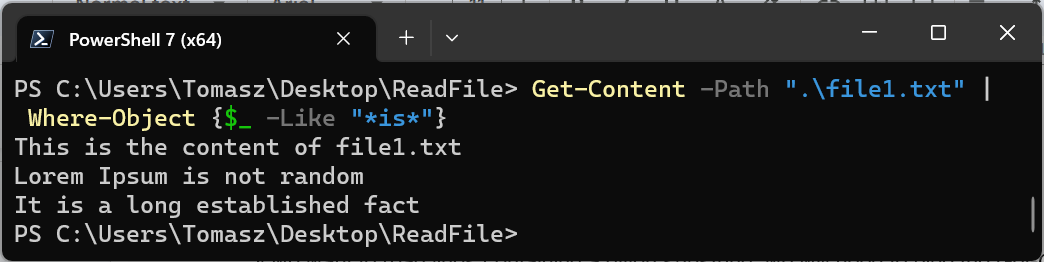
Example 6: Getting line numbers with a specific substring
This modifies Example 5. Instead of getting the lines with a given substring, we want their line numbers.
1 |
Get-Content -Path ".\file1.txt" | Select-String -Pattern "is" -AllMatches | Select-Object -ExpandProperty LineNumber # Output 1, 2 and 4 |
Note that Select-String also supports regular expressions.
Example 7: Loading contents of a file as a single string
This is done using the -Raw parameter.
1 2 |
$data = Get-Content -Path ".\file1.txt" -Raw $data.Count # 1 |
When the -Raw parameter is passed, the array is returned by Get-Content as one element as shown by $data.Count
Example 8: Remove black lines
In this case, we need to use the Where-Object cmdlet and Trim() function to filter out empty and blank lines, as shown below.
1 |
Get-Content -Path ".\file1.txt" | Where-Object {$_.Trim()} |
Example 9: Looping through the contents of the file line by line
This example shows how to use a for-loop to process the file line by line.
1 2 3 4 |
$data = Get-Content -Path ".\file1.txt" ForEach ($row in $data){ Write-Host $row -Replace "t", "zzz" } |
The code snippet above loads the file1.txt file using Get-Content and loops through each file line, replacing the letter “t” with “zzz”.
Example 10: Using filters to load contents from several files
We need to issue filters on the files using the -Filter parameter. The following code example shows how to load all text files in the current working directory, excluding files starting with “misc”.
1 |
$data = Get-Content -Path "./*" -Filter "*.txt" -Exclude "misc*" |
Example 11: Loading CSV using Import-Csv
This example loads the employees.csv file introduced at the start of the article. The file contains 2 files – name and id – and 2 records. Values on each record are separated by “|” (the -Delimiter).
1 2 |
$data = Get-Content -Path ".\employees.csv" -Delimiter "|" $data |
You can then index the values of $data just like we did for Get-Content in Example 2
Conclusion
This article discussed how to use Get-Content and Import-Csv to load file contents into a PowerShell array. Through 11 examples, we covered different used cases for the two cmdlets.