This article discusses two ways of saving PowerShell output into a variable. In each method, we will work on examples to cement the ideas.
Method 1: Using the Assignment Operator (=)
The most common way to save the output of a PowerShell command into a variable is using:
1 |
$var = <command> |
Now, you can use the $var variable to perform further operations on the output of the <command>.
1 2 3 4 5 |
# Save an array of 5 elements into a variable <br>$var1 = 1, 2, 4, -5, 9 # Display the array by calling the variable $var1 # Count the number of elements in the array $var1.Count # Output: 5 |
Note: when the <command> runs successfully without an error, the output is sent to the success stream (stream #1), and if the <command> execution ends in error, the result is streamed to the error stream (stream #2).
In the $var = <command> line, the $var variable only picks data from the success stream (not error steam). In the example below, Get-ChildItem attempts to get child items from a folder that doesn’t exist, leading to an error. Notice that the variable $var2 does not pick the error; instead, the error output is sent to the console.
1 2 |
$var2 = Get-ChildItem "./test_folder" $var 2 |

If you want also to pick the error output on the variable, use the following line to redirect the output.
1 |
$var = <command> 2>&1 |
The 2>&1 means we want to redirect the content of the error stream (Stream #2) to the success stream (Stream #1), which can now be stored on the variable.

The example above shows that the error message has now been captured in the variable $var2.
Note: the redirection command given above only works from within PowerShell. If you run an external command, you may need to modify the command slightly – enclose 2>&1 in quotes, that is
1 |
$var = <command> "2>&1" |
For example, the following command runs the dir using cmd.exe (not PowerShell).
1 2 |
$var2 = cmd /c dir "Desktop1" "2>&1" $var2 |
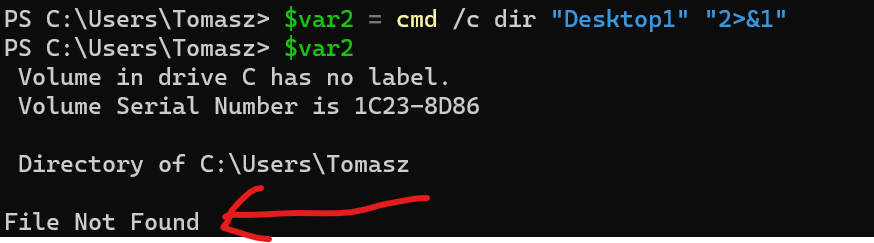
The assignment operator can also be used with chained commands, as shown below. In this case, the output of <command-3> is saved on the $var
1 |
$var = <command-1> | <command-2> | <command-3> |
Example:
1 2 |
$var2 = 3, 4, 5, 6, -6 | Sort-Object | Select-Object -First 1 $var2 |

Method 2: Using the -OutVariable or -Ov Parameter
Note that in Method 1, when a variable captures command output, the output is not printed to the console unless you run the variable name as a command.
This is where this method comes in. The -OutVariable parameter allows us to save the data in a variable while letting it get printed on the console.
You can capture the output to a variable using the following syntax:
1 2 |
<command> -OutVariable var_name $var_name |
For chained commands, you will use this
1 2 |
<command-1> | <command-2> -OutVariable var_name $var_name |
For example,
1 2 |
Write-Output "Hello World" |Select-Object Length -OutVariable var5 $var5 |
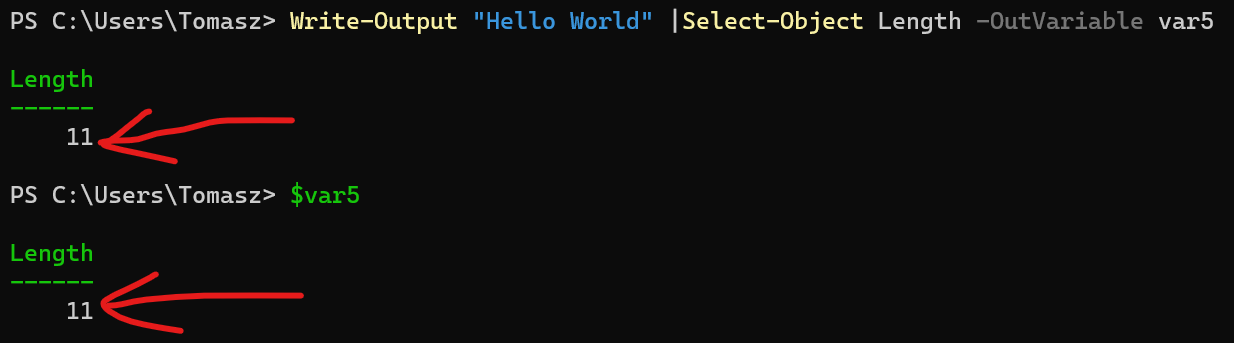
The code above takes the “Hello World” string, gets its length, and saves the output on the $var5 variable.
Conclusion
This guide discussed two methods for saving PowerShell output into a variable. The first method uses the assignment operator (=), and the second method uses the -OutVariable parameter. In most cases, Method 1 does the job. The second method is handy if you want to save your data in a variable while still letting it get printed on the console.