You can create a Python file directly from the terminal. Follow these steps to do it:
- Open Command Prompt (cmd).
- Open the Python interpreter by typing python inside the terminal.
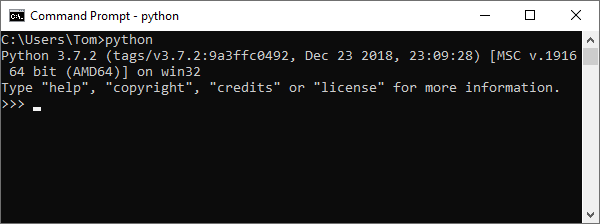
- Open (or create if it doesn’t exist) a new file for writing inside an existing directory.
1 |
my_file = open("D:/temp/python_file.py", "w+") |
- The file is created. Now, you can write to it. Create a loop:
1 |
for i in range(6): |
- Add a text you want to repeat. Remember to add an indentation at the beginning.
1 |
my_file.write("This is line number %d\n." % (i+1)) |
- It’s a good practice to close the file after the process:
1 |
my_file.close() |
The full code:
1 2 3 4 5 6 |
my_file = open("D:/temp/python_file.py", "w+") for i in range(6): my_file.write("This is line number %d." % (i + 1)) my_file.close() |