The dunder __file__ is a variable that stores the path to the Python module/script being imported (executed). It is generated when the actual module or script is executed.
For example, if we save the following print statement on test1.py and execute it, we get the output as shown.
1 |
print(__file__) |
Output:
/home/kiprono/Desktop/ToT/test1.py
As shown in the output, the __file__ variable holds the full path of the file being executed.
What Causes the “__file__ is not defined” Error?
The error is raised when we execute a Python script/module on the interactive shell. This is because the __file__ variable is not defined in such a context.
Example 1: Using Python interactive shell in the command line
The first sure way to get the “__file__ is not defined” error is by executing code with the __file__ variable from the interactive shell. An example is shown in the figure below.
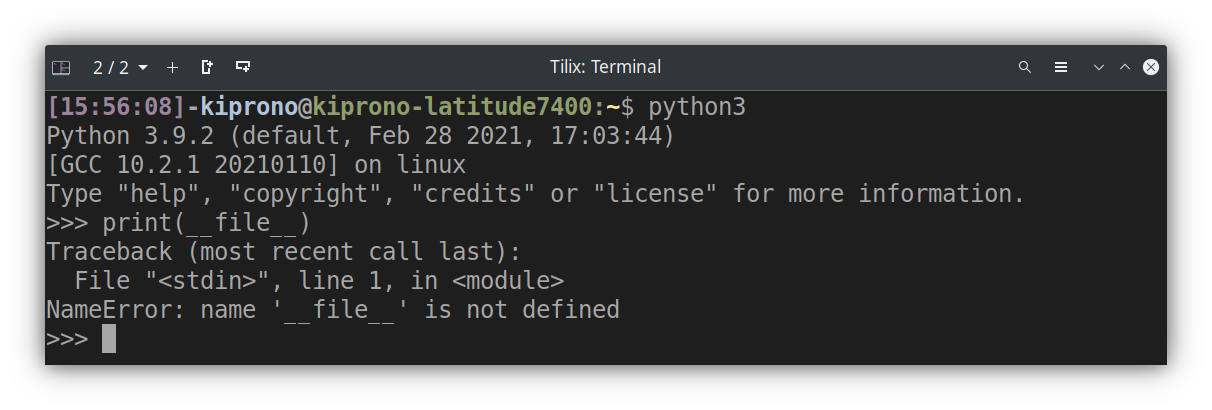
Example 2: Calling __file__ dunder on JuPyter notebook
JuPyter notebook runs on the Interactive shell under the hoods. That is why using the magic variable __file__ to get the path of the notebook being executed ends in a “__file__ is not defined” error.
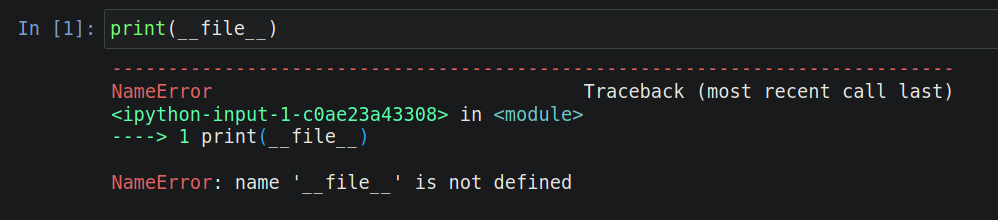
Final take on reproducing the error: If you run a script/module outside an interactive environment, you shouldn’t get the “__file__ is not defined” error. However, if you must run your code on JuPyter notebooks or any other coding platform calling the interactive shell, you need to explore the solutions discussed below.
Solutions to “__file__ is not defined” in Python
This section covers four solutions to the error. These solutions provide alternatives to using the __file__ variable.
Solution 1: Using the os.getcwd() function
The os.getcwd() function returns the current working directory – the location of the script being executed.
1 2 |
import os print(os.getcwd()) |
Output (Depends on the location of the script you are executing):
/home/kiprono/Desktop/ToT
If you want to get the full path of a JuPyter notebook, you can use the os.getcwd() function with some JavaScript code, as shown below.
1 2 3 4 5 |
%%javascript # this is not a comment. Include it in the code. IPython.notebook.kernel.execute('notebook_name = "' + IPython.notebook.notebook_name + '"') import os nb_full_path = os.path.join(os.getcwd(), notebook_name) nb_full_path |

Solution 2: Using os.path.abspath(<path>) function
You can also get the current working directory using os.path.abspath(<path>) passing an empty string as a path.
1 2 3 |
import os path1 = os.path.abspath("") print(path1) |
Solution 3: Getting file path in the global variables
In most cases, global variables should contain traces for the path to the current file. If you call the globals() function, you will get a dictionary with paths and variables used in the script.
For the case of getting the current working directory, you can use the value with the “_dh” key (make sure to view all items on global() to ascertain that it is the correct key).
1 2 3 4 5 6 |
# Viewing all items vars1 = globals() print(vars1) # Accessing the current working directory. cwd = globals()["_dh"][0] print(cwd) |
Output (truncated):
{'__name__': '__main__', …, '_oh': {2: '/home/kiprono/Desktop/ToT'}, '_dh': ['/home/kiprono/Desktop/ToT'], 'In': ['', 'print(__file__)', 'import os\nos.path.abspath("")', 'this_path = os.path.abspath(os.path.dirname(__file__))\nprint(this_path)', '# Viewing all items\nvars1 = globals()\nprint(vars1)\n\n# Accessing the current working directory.\ncwd = globals()["_dh"][0]\nprint(cwd)'], …}
/home/kiprono/Desktop/ToT
Solution 4: Using the pathlib package
1 2 3 4 5 6 |
from pathlib import Path cwd = Path().resolve() print(cwd) # or this cwd = Path.cwd() print(cwd) |
Output:
/home/kiprono/Desktop/ToT /home/kiprono/Desktop/ToT
Conclusion
The “__file__ is not defined” error is raised when you call the __file__ variable in a Python interactive environment. You can run the code outside the interactive shell or use the three solutions suggested in this article to solve the error.