Slicing a list of lists in Python can be done in three main ways:
- Method 1: Using list comprehension,
- Method 2: Using for-loop and,
- Method 3: Using NumPy
Let’s go through them.
Method 1: Using List Comprehension
This approach allows us to slice the main list and the sublists even if the sublists are not of the same length.
The general syntax for list comprehension for slicing is given in the Figure below.
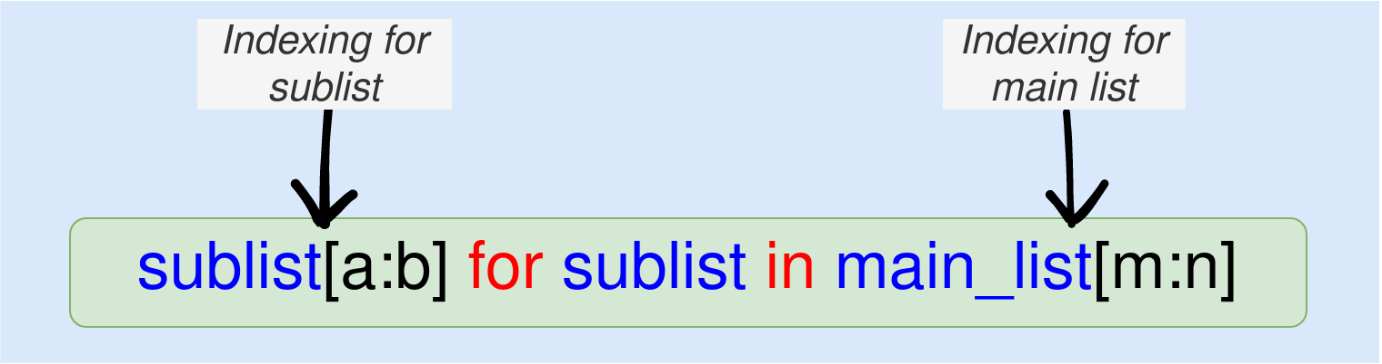
Here are some examples in code.
1 2 3 4 5 6 7 8 9 10 11 |
# A list within a list - the main list lst1 = [["Sam", 96, "Warsaw", None], ["Belinda", 78, "Israel", "GT"], ["Sally", 56, "SA", "SL"], ["Smith", 77, "Poland", "SZ"]] # Get the second and third elements of each sublist for the third sublist to the end lst2 = [sublist[1:3] for sublist in lst1[2:]] print("lst2: ", lst2) # Get the first three elements of each sublist for all sublists in the main list lst3 = [sublist[:3] for sublist in lst1] print("lst3: ", lst3) # Get the last two elements of the sublists for the first three sublists. lst4 = [sublist[-2:] for sublist in lst1[:3]] print("lst4: ", lst4) |
Output:
lst2: [[56, 'SA'], [77, 'Poland']] lst3: [['Sam', 96, 'Warsaw'], ['Belinda', 78, 'Israel'], ['Sally', 56, 'SA'], ['Smith', 77, 'Poland']] lst4: [['Warsaw', None], ['Israel', 'GT'], ['SA', 'SL']]
Method 2: Using for-loop
This method iterates through sublists on the main lists and elements within the sublist and picks the selected elements.
Here is an example of picking the first three elements for each second and the third sublist.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
lst1 = [ ["Sam", 96, "Warsaw", None], ["Belinda", 78, "Israel", "GT"], ["Sally", 56, "SA", "SL"], ["Smith", 77, "Poland", "SZ"]] # Initialize a list to hold the sliced main list. derived_list = [] # Pick elements from the second and third sublists for sublist in lst1[1:3]: # An empty list to hold slice sublists derived_sublist = [] # Get the second to the last element of the sublists for item in sublist[:3]: # Append element of selected sublists to derived_sublist derived_sublist.append(item) # Append the picked sublists to the main list. derived_list.append(derived_sublist) print(derived_list) |
Output:
[['Belinda', 78, 'Israel'], ['Sally', 56, 'SA']]
Method 3: Using NumPy
This is a very efficient method if your sublists are the same length. The following Figure shows the general syntax for slicing a list of lists in Python using NumPy.
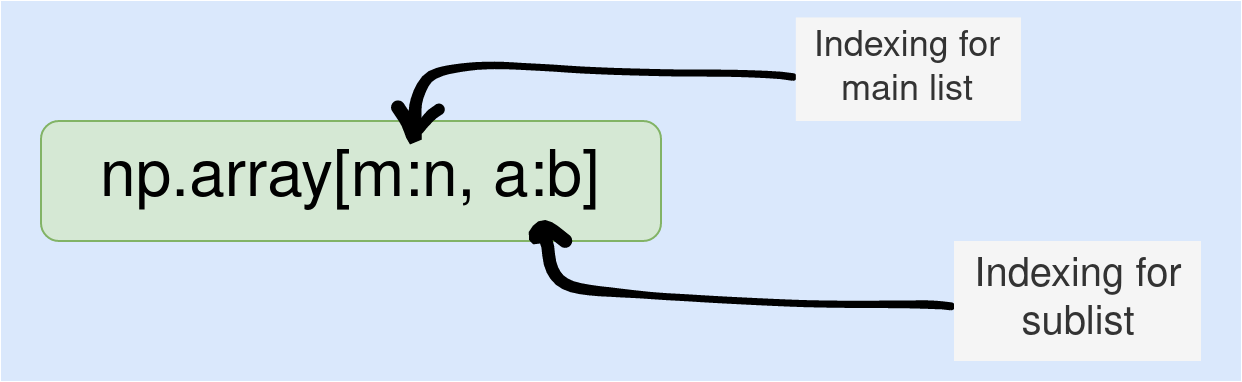
Here are some examples of implementing slicing with NumPy
1 2 3 4 5 6 7 8 9 10 11 12 13 |
import numpy as np lst1 = [["Sam", 96, "Warsaw", None], ["Belinda", 78, "Israel", "GT"], ["Sally", 56, "SA", "SL"], ["Smith", 77, "Poland", "SZ"]] # Convert the list of lists into a numpy array arr1 = np.array(lst1) # Slice the main list and cast the result into the list # arr1[:,:2] picks the first two elements for all sublists lst2 = arr1[:, :2].tolist() print("lst2: ", lst2) # arr1[1:3, 2:] gets the second and the third elements of sublists for # second sublist to the end. lst3 = arr1[1:3, 2:].tolist() print("lst3: ", lst3) |
Output:
lst2: [['Sam', 96], ['Belinda', 78], ['Sally', 56], ['Smith', 77]] lst3: [['Israel', 'GT'], ['SA', 'SL']]
Conclusion
This article discussed three methods for slicing nested lists in Python. The list comprehension and for-loop methods work for a nested list with sublists of different lengths, but the NumPy method needs sublists to be of the same sizes.